elegoo uno manual
- by chaya
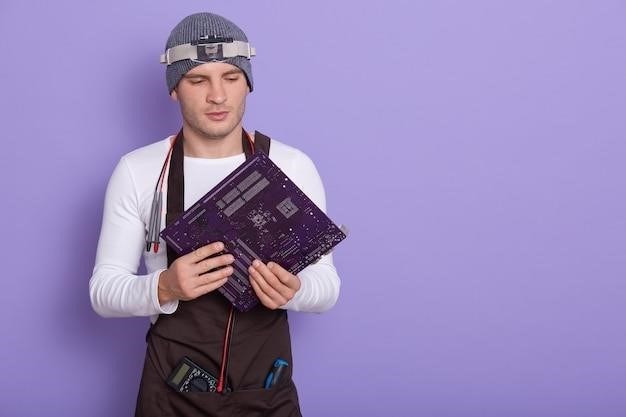
Elegoo UNO R3⁚ A Comprehensive Guide
This guide provides a complete walkthrough of the Elegoo UNO R3, covering setup, programming basics, and advanced projects. Explore tutorials, code examples, and troubleshooting tips for a smooth learning experience. Numerous online resources and community support are available.
Getting Started with the Elegoo UNO R3 Starter Kit
The Elegoo UNO R3 Starter Kit is a popular choice for beginners venturing into the world of Arduino programming. This comprehensive kit typically includes the Elegoo UNO R3 board itself – a microcontroller board compatible with the Arduino IDE – along with a variety of components crucial for building various projects. These components often encompass LEDs of different colors (including RGB LEDs for more complex lighting effects), resistors to control current flow, potentiometers for variable resistance, buttons for user input, and various sensors such as photoresistors or temperature sensors. The kit frequently provides connecting wires (often jumper wires), a breadboard for prototyping circuits, and a USB cable for connecting the board to your computer. The inclusion of a detailed tutorial or manual is a key feature, guiding users through the setup process and providing step-by-step instructions for a range of projects, fostering a practical, hands-on learning experience. Some kits even integrate a power supply module or a 9V battery for convenient power delivery. This all-in-one approach simplifies the initial investment and makes the learning process much smoother for newcomers. The kit’s compatibility with the Arduino IDE ensures a user-friendly programming environment, making it an ideal entry point for electronics enthusiasts and budding programmers.
Installing the Arduino IDE and Necessary Software
Before embarking on your Elegoo UNO R3 projects, you must install the Arduino Integrated Development Environment (IDE). This software is the cornerstone of your Arduino programming journey. Download the latest version of the Arduino IDE from the official Arduino website, ensuring compatibility with your operating system (Windows, macOS, or Linux). The installation process is typically straightforward, involving a simple executable file download and execution. Once installed, you may need to install the appropriate board support packages. This step ensures the IDE recognizes your Elegoo UNO R3 board. Navigate to the “Tools” menu within the IDE, select “Board,” and find the “Arduino/Genuino Uno” option within the available boards. If it isn’t present, you’ll need to use the “Boards Manager” to install it, searching for “Arduino AVR Boards” and completing the installation process. This step is crucial as it provides the necessary compilers and libraries for your code to function correctly. After successful installation, connect your Elegoo UNO R3 to your computer via the USB cable, and the IDE should detect the board’s COM port. Select the correct COM port from the “Tools” menu, ensuring proper communication between your computer and the microcontroller. With the IDE and necessary drivers installed, you are ready to begin your first Arduino program. Remember that additional libraries may be needed for specific sensors or components used in more advanced projects.
Understanding the Elegoo UNO R3 Board and its Components
The Elegoo UNO R3 is a microcontroller board based on the ATmega328P, a powerful and versatile chip. Familiarize yourself with its key components⁚ the USB port for connecting to your computer, providing power and communication; the power jack for external power supply using a 9V battery or wall adapter; digital input/output (I/O) pins, offering both input and output capabilities for controlling various components; analog input pins, essential for reading data from analog sensors like potentiometers and light sensors; a reset button, used to restart the board; the power LED, indicating whether the board is receiving power; and the RX and TX LEDs, showing communication with your computer. The board also includes a crystal oscillator for precise timing, crucial for many applications. Understanding the pin layout is crucial for connecting components correctly; Refer to the official Elegoo UNO R3 schematic or the provided documentation for a detailed pinout diagram. Many online resources offer detailed explanations and visuals of the board’s components, including interactive schematics and pinout diagrams; Properly identifying and understanding each part will allow for seamless integration with components and a smooth programming experience. The board’s open-source nature allows for extensive customization and community support.
Connecting the Elegoo UNO R3 to Your Computer
Connecting your Elegoo UNO R3 to your computer is the first step in your Arduino journey. Use the included USB cable to connect the board’s USB port to an available USB port on your computer. Before powering on, ensure the Arduino IDE is installed and configured correctly. The IDE will automatically detect the connected board upon successful connection. If not detected, check the USB cable for any damage and ensure it’s securely plugged into both the board and your computer. You may need to install the correct drivers for your operating system; these are usually included with the Arduino IDE installer. Windows users might need to check the Device Manager for any errors or unknown devices. Sometimes, changing USB ports can resolve connection issues. After successfully connecting, the power LED on the board should illuminate, indicating power is being supplied through the USB connection. The board’s COM port will be displayed within the Arduino IDE’s “Tools” menu under “Port.” This COM port identifies the serial communication pathway between your computer and the board, necessary for uploading code and monitoring data. If you encounter errors, review your connections, driver installation, and ensure the correct COM port is selected in the IDE. Online tutorials and troubleshooting guides are readily available to assist in resolving connection problems. Once the connection is established, you are ready to start programming your Elegoo UNO R3.
Basic Programming Concepts for Arduino UNO R3
The Arduino UNO R3 utilizes a simplified C++-based programming language, making it accessible even for beginners. Central to Arduino programming are the setup
and loop
functions. setup
executes only once at the start of the program, initializing variables, setting pin modes (INPUT or OUTPUT), and configuring serial communication. loop
runs repeatedly after setup
is complete, forming the main control flow of your program. You’ll interact extensively with digital and analog I/O pins. Digital pins control high/low voltage states (ON/OFF), ideal for LEDs and switches. Analog pins read variable voltages, providing data from sensors like potentiometers or light-dependent resistors (LDRs). Variables store data values. Constants are fixed values, improving code readability and maintainability. Comments (using //
for single-line and /* ... /
for multi-line) enhance code understanding. Serial communication enables sending data to and receiving data from your computer, facilitating debugging and interaction with your programs. Operators perform actions on variables (e.g., +, -, , /, =). Conditional statements (if
, else if
, else
) control program flow based on conditions. Loops (for
, while
) repeat blocks of code until a condition is met. Functions organize code into reusable blocks, improving program structure.
The Blink Project⁚ Your First Arduino Program
The “Blink” project is the quintessential introductory Arduino program. It demonstrates basic I/O control by repeatedly turning an LED on and off. This seemingly simple program introduces fundamental concepts vital to more complex projects. First, you’ll need an LED and a 220-ohm resistor. Connect the longer (positive) leg of the LED to pin 13 of the Elegoo UNO R3 through the resistor, and the shorter (negative) leg to ground. The Arduino IDE provides a user-friendly interface for writing and uploading code. Open the IDE, create a new sketch (File > New), and enter the following code⁚ void setup { pinMode(13, OUTPUT); } void loop { digitalWrite(13, HIGH); delay(1000); digitalWrite(13, LOW); delay(1000); }
. This code sets pin 13 as an output, then repeatedly sets it HIGH (LED on), waits one second (delay(1000)
), sets it LOW (LED off), and waits another second. After saving the code (File > Save As), select your board and port (Tools > Board, Tools > Port), then click the upload button (the right-pointing arrow). Observe the LED blinking—your first successful Arduino program! This simple example lays the foundation for understanding digital I/O control, timing functions (delay
), and the basic structure of Arduino programs (setup
and loop
). Understanding this project unlocks the potential for creating a wide array of interactive projects.
Working with LEDs and Digital Inputs/Outputs
Expanding on the Blink project, this section delves into the versatile world of LEDs and digital I/O pins on the Elegoo UNO R3. Understanding digital pins is crucial for interacting with a wide range of components. These pins operate in a binary fashion⁚ either HIGH (5V) or LOW (0V), perfectly suited for controlling LEDs. Remember to always use a current-limiting resistor (typically 220 ohms for standard LEDs) to prevent damage. Experiment with different pin configurations to control multiple LEDs independently. The Arduino IDE simplifies this process; the digitalWrite
function sets a pin’s state, while pinMode
configures a pin as either INPUT or OUTPUT. Explore the concept of digital inputs by connecting a button to a digital pin. When the button is pressed, it connects the pin to ground, registering a LOW signal; otherwise, it’s HIGH. This allows your program to respond to user interactions. The digitalRead
function retrieves the state of a digital input pin. Incorporate this into your code to create interactive projects. Combine LEDs and buttons to create simple on/off switches or more intricate patterns. Mastering LEDs and digital I/O opens the door to a vast range of possibilities, from basic circuits to complex interactive systems. Don’t hesitate to explore various circuit configurations and experiment with the Arduino IDE’s capabilities. The Elegoo UNO R3’s documentation provides valuable insights and examples to further enhance your understanding.
Utilizing Analog Inputs and Sensors
The Elegoo UNO R3 boasts six analog input pins, expanding your project capabilities beyond simple on/off controls. Unlike digital pins, analog inputs read varying voltage levels, providing a range of values (0-1023) representing the signal strength. This feature enables the integration of sensors that output analog signals, such as potentiometers, light-dependent resistors (LDRs), and temperature sensors. A potentiometer allows for variable voltage control, providing a smooth adjustment rather than a binary HIGH/LOW state. Connecting a potentiometer to an analog pin and reading its value with the analogRead
function allows you to control the brightness of an LED or the speed of a motor. LDRs measure ambient light intensity, providing a variable voltage based on light levels. This opens doors for light-sensitive projects, such as automated lighting systems or light-dependent music players. Temperature sensors, such as thermistors, offer analog voltage output correlated to temperature readings. Connect a thermistor and use analogRead
to monitor temperature and trigger actions accordingly. Remember, understanding the sensor’s datasheet and appropriate scaling is key to accurately interpreting the data. The Arduino IDE simplifies this process, providing examples and libraries to facilitate integration. Elegoo’s comprehensive tutorials and online resources offer extensive guidance on working with analog inputs and various sensors, enabling you to create innovative and responsive projects. Explore the diverse functionalities of analog inputs to unlock the full potential of your Elegoo UNO R3.
Advanced Projects and Tutorials
Having mastered the fundamentals, delve into more complex projects using your Elegoo UNO R3. Elegoo provides numerous advanced tutorials and project ideas to expand your skills. These projects often involve multiple sensors, actuators, and more sophisticated programming techniques. Consider building a robot car, incorporating motor control, ultrasonic sensors for obstacle avoidance, and potentially even a camera module for image processing. A weather station project could integrate temperature, humidity, and pressure sensors, displaying the data on an LCD screen or transmitting it wirelessly. For those interested in home automation, explore projects involving controlling lights, appliances, or security systems using relays and sensor feedback. More ambitious projects could involve interfacing with external displays like TFT screens, creating custom interfaces and user experiences. The wealth of online resources, including Elegoo’s extensive documentation and the broader Arduino community, provides ample support for tackling these challenges. Advanced projects demand a deeper understanding of programming concepts such as interrupts, state machines, and data manipulation. Remember to thoroughly test and debug your code at each step, using the serial monitor for valuable feedback. Don’t hesitate to explore online forums and communities for assistance when facing roadblocks. With dedication and persistence, the advanced projects will greatly enhance your understanding of embedded systems and Arduino programming.
Troubleshooting Common Issues with Elegoo UNO R3
Encountering problems with your Elegoo UNO R3 is a common part of the learning process. One frequent issue is the board failing to upload code. This can stem from incorrect port selection in the Arduino IDE, ensuring the correct COM port is chosen for your board. Double-check the connections between your computer and the UNO R3, ensuring the USB cable is securely plugged into both. Another potential cause is a corrupted IDE installation; reinstalling the software can resolve this. If the board still refuses to upload, examine the power supply; insufficient power can prevent proper operation. The LED lights on the board can provide clues; a lack of power may be indicated by their absence. Problems with code often arise from simple syntax errors, so carefully review your code for typos, missing semicolons, or incorrect function calls. The Arduino IDE’s error messages can often pinpoint the problem’s location. If your code compiles but the desired behavior isn’t observed, check your wiring diagrams meticulously. Loose connections, incorrect pin assignments, or damaged components are common culprits. Always use a multimeter to verify the voltage and current levels at different points in your circuit. The Elegoo UNO R3 has a resettable fuse to protect against overcurrent; if this fuse blows, you might need to replace it. Online forums and the Elegoo support documentation provide a wealth of troubleshooting resources and community-based solutions, addressing many common problems encountered with the Elegoo UNO R3.
Exploring Elegoo’s Additional Resources and Support
Elegoo offers a robust ecosystem of support resources to complement your Elegoo UNO R3 experience. Beyond the basic manual, delve into their extensive online documentation, which provides in-depth tutorials, code examples, and troubleshooting guides for various projects. These resources cater to all skill levels, from beginners to experienced users. The Elegoo website often features blog posts, articles, and video tutorials that provide step-by-step instructions and explanations for common tasks and advanced projects. This ensures that users have access to clear and concise information. Furthermore, Elegoo maintains an active online community forum where users can interact, share their projects, ask questions, and assist one another. This provides a valuable platform for peer-to-peer support and collaborative learning. Engaging with this community can often provide quick solutions to problems and foster a sense of camaraderie among users. Elegoo’s customer support team is also readily available to address specific inquiries or technical issues. They can provide direct assistance, guiding users through complex problems or offering tailored solutions for unique situations. Don’t hesitate to contact them; their expertise can be invaluable in overcoming challenges. Remember to check the Elegoo website for announcements regarding software updates, new features, or additional learning materials that may enhance your experience with the Elegoo UNO R3. By leveraging these resources, you can unlock the full potential of your kit and embark on a rewarding journey of exploration and innovation.
Unlock the power of your Elegoo Uno R3 with our comprehensive manual! From beginner basics to advanced projects, we’ve got you covered. Dive into coding, circuit building, and unleash your creativity. Get started today!